
The 2.4 inch TFT Touch Screen Module with micro SD card slot is now available as a SHIELD for Arduino UNO. It has a four wire resistive touch screen, a micro SD card socket, a reset switch and a convenient Arduino Uno shield footprint.
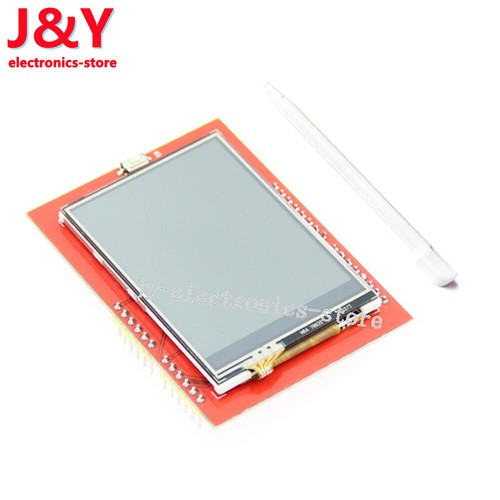
In this tutorial, you will learn how to use and set up 2.4″ Touch LCD Shield for Arduino. First, you’ll see some general information about this shield. And after learning how to set the shield up, you’ll see 3 practical projects.
The role of screens in electronic projects is very important. Screens can be of very simple types such as 7 Segment or character LCDs or more advanced models like OLEDs and TFT LCDs.
One of the most important features of this LCD is including a touch panel. If you are about to use the LCD, you need to know the coordinates of the point you touch. To do so, you should upload the following code on your Arduino board and open the serial monitor. Then touch your desired location and write the coordinates displayed on the serial monitor. You can use this coordination in any other project./*TFT LCD - TFT Touch CoordinateBased on Librery Examplemodified on 21 Feb 2019by Saeed Hosseinihttps://electropeak.com/learn/*/#include #include "TouchScreen.h"#define YP A2#define XM A3#define YM 8#define XP 9// For better pressure precision, we need to know the resistance// between X+ and X- Use any multimeter to read it// For the one we"re using, its 300 ohms across the X plateTouchScreen ts = TouchScreen(XP, YP, XM, YM, 300);void setup(void) {Serial.begin(9600);}void loop(void) {TSPoint p = ts.getPoint();if (p.z > ts.pressureThreshhold) {Serial.print("X = "); Serial.print(p.x);Serial.print("\tY = "); Serial.print(p.y);Serial.print("\tPressure = "); Serial.println(p.z);}delay(100);}
Displaying Text and Shapes on Arduino 2.4 LCD/*TFT LCD - TFT Simple drivingmodified on 21 Feb 2019by Saeed Hosseinihttps://electropeak.com/learn/*/#include #include #define LCD_CS A3#define LCD_CD A2#define LCD_WR A1#define LCD_RD A0#define LCD_RESET A4#define BLACK 0x0000#define BLUE 0x001F#define RED 0xF800#define GREEN 0x07E0#define CYAN 0x07FF#define MAGENTA 0xF81F#define YELLOW 0xFFE0#define WHITE 0xFFFF#define ORANGE 0xFD20#define GREENYELLOW 0xAFE5#define NAVY 0x000F#define DARKGREEN 0x03E0#define DARKCYAN 0x03EF#define MAROON 0x7800#define PURPLE 0x780F#define OLIVE 0x7BE0#define LIGHTGREY 0xC618#define DARKGREY 0x7BEFAdafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET);void setup() {Serial.begin(9600);Serial.println(F("TFT LCD test"));#ifdef USE_ADAFRUIT_SHIELD_PINOUTSerial.println(F("Using Adafruit 2.4\" TFT Arduino Shield Pinout"));#elseSerial.println(F("Using Adafruit 2.4\" TFT Breakout Board Pinout"));#endifSerial.print("TFT size is ");Serial.print(tft.width());Serial.print("x");Serial.println(tft.height());tft.reset();uint16_t identifier = tft.readID();if (identifier == 0x9325) {Serial.println(F("Found ILI9325 LCD driver"));} else if (identifier == 0x9328) {Serial.println(F("Found ILI9328 LCD driver"));} else if (identifier == 0x7575) {Serial.println(F("Found HX8347G LCD driver"));} else if (identifier == 0x9341) {Serial.println(F("Found ILI9341 LCD driver"));} else if (identifier == 0x8357) {Serial.println(F("Found HX8357D LCD driver"));} else {Serial.print(F("Unknown LCD driver chip: "));Serial.println(identifier, HEX);Serial.println(F("If using the Adafruit 2.4\" TFT Arduino shield, the line:"));Serial.println(F(" #define USE_ADAFRUIT_SHIELD_PINOUT"));Serial.println(F("should appear in the library header (Adafruit_TFT.h)."));Serial.println(F("If using the breakout board, it should NOT be #defined!"));Serial.println(F("Also if using the breakout, double-check that all wiring"));Serial.println(F("matches the tutorial."));return;}tft.begin(identifier);Serial.println(F("Benchmark Time (microseconds)"));Serial.print(F("Screen fill "));Serial.println(FillScreen());delay(500);tft.setTextColor(YELLOW);tft.setCursor(70, 180);tft.setTextSize(1);tft.println("Electropeak");delay(200);tft.fillScreen(PURPLE);tft.setCursor(50, 170);tft.setTextSize(2);tft.println("Electropeak");delay(200);tft.fillScreen(PURPLE);tft.setCursor(20, 160);tft.setTextSize(3);tft.println("Electropeak");delay(500);tft.fillScreen(PURPLE);for (int rotation = 0; rotation < 4; rotation++) { tft.setRotation(rotation); tft.setCursor(0, 0); tft.setTextSize(3); tft.println("Electropeak"); delay(700); } delay(500); Serial.print(F("Rectangles (filled) ")); Serial.println(testFilledRects(YELLOW, MAGENTA)); delay(500); } void loop() { } unsigned long FillScreen() { unsigned long start = micros(); tft.fillScreen(RED); delay(500); tft.fillScreen(GREEN); delay(500); tft.fillScreen(BLUE); delay(500); tft.fillScreen(WHITE); delay(500); tft.fillScreen(MAGENTA); delay(500); tft.fillScreen(PURPLE); delay(500); return micros() - start; } unsigned long testFilledRects(uint16_t color1, uint16_t color2) { unsigned long start, t = 0; int n, i, i2, cx = tft.width() / 2 - 1, cy = tft.height() / 2 - 1; tft.fillScreen(BLACK); n = min(tft.width(), tft.height()); for (i = n; i > 0; i -= 6) {i2 = i / 2;start = micros();tft.fillRect(cx - i2, cy - i2, i, i, color1);t += micros() - start;// Outlines are not included in timing resultstft.drawRect(cx - i2, cy - i2, i, i, color2);}return t;}
Displaying BMP pictures/*This code is TFTLCD Library Example*/#include #include #include #include #define LCD_CS A3#define LCD_CD A2#define LCD_WR A1#define LCD_RD A0#define SD_CS 10Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, A4);void setup(){Serial.begin(9600);tft.reset();uint16_t identifier = tft.readID();if (identifier == 0x9325) {Serial.println(F("Found ILI9325 LCD driver"));} else if (identifier == 0x9328) {Serial.println(F("Found ILI9328 LCD driver"));} else if (identifier == 0x7575) {Serial.println(F("Found HX8347G LCD driver"));} else if (identifier == 0x9341) {Serial.println(F("Found ILI9341 LCD driver"));} else if (identifier == 0x8357) {Serial.println(F("Found HX8357D LCD driver"));} else {Serial.print(F("Unknown LCD driver chip: "));Serial.println(identifier, HEX);Serial.println(F("If using the Adafruit 2.4\" TFT Arduino shield, the line:"));Serial.println(F(" #define USE_ADAFRUIT_SHIELD_PINOUT"));Serial.println(F("should appear in the library header (Adafruit_TFT.h)."));Serial.println(F("If using the breakout board, it should NOT be #defined!"));Serial.println(F("Also if using the breakout, double-check that all wiring"));Serial.println(F("matches the tutorial."));return;}tft.begin(identifier);Serial.print(F("Initializing SD card..."));if (!SD.begin(SD_CS)) {Serial.println(F("failed!"));return;}Serial.println(F("OK!"));bmpDraw("pic1.bmp", 0, 0);delay(1000);bmpDraw("pic2.bmp", 0, 0);delay(1000);bmpDraw("pic3.bmp", 0, 0);delay(1000);}void loop(){}#define BUFFPIXEL 20void bmpDraw(char *filename, int x, int y) {File bmpFile;int bmpWidth, bmpHeight; // W+H in pixelsuint8_t bmpDepth; // Bit depth (currently must be 24)uint32_t bmpImageoffset; // Start of image data in fileuint32_t rowSize; // Not always = bmpWidth; may have paddinguint8_t sdbuffer[3 * BUFFPIXEL]; // pixel in buffer (R+G+B per pixel)uint16_t lcdbuffer[BUFFPIXEL]; // pixel out buffer (16-bit per pixel)uint8_t buffidx = sizeof(sdbuffer); // Current position in sdbufferboolean goodBmp = false; // Set to true on valid header parseboolean flip = true; // BMP is stored bottom-to-topint w, h, row, col;uint8_t r, g, b;uint32_t pos = 0, startTime = millis();uint8_t lcdidx = 0;boolean first = true;if ((x >= tft.width()) || (y >= tft.height())) return;Serial.println();Serial.print(F("Loading image ""));Serial.print(filename);Serial.println("\"");// Open requested file on SD cardif ((bmpFile = SD.open(filename)) == NULL) {Serial.println(F("File not found"));return;}// Parse BMP headerif (read16(bmpFile) == 0x4D42) { // BMP signatureSerial.println(F("File size: ")); Serial.println(read32(bmpFile));(void)read32(bmpFile); // Read & ignore creator bytesbmpImageoffset = read32(bmpFile); // Start of image dataSerial.print(F("Image Offset: ")); Serial.println(bmpImageoffset, DEC);// Read DIB headerSerial.print(F("Header size: ")); Serial.println(read32(bmpFile));bmpWidth = read32(bmpFile);bmpHeight = read32(bmpFile);if (read16(bmpFile) == 1) { // # planes -- must be "1"bmpDepth = read16(bmpFile); // bits per pixelSerial.print(F("Bit Depth: ")); Serial.println(bmpDepth);if ((bmpDepth == 24) && (read32(bmpFile) == 0)) { // 0 = uncompressedgoodBmp = true; // Supported BMP format -- proceed!Serial.print(F("Image size: "));Serial.print(bmpWidth);Serial.print("x");Serial.println(bmpHeight);// BMP rows are padded (if needed) to 4-byte boundaryrowSize = (bmpWidth * 3 + 3) & ~3;// If bmpHeight is negative, image is in top-down order.// This is not canon but has been observed in the wild.if (bmpHeight < 0) { bmpHeight = -bmpHeight; flip = false; } // Crop area to be loaded w = bmpWidth; h = bmpHeight; if ((x + w - 1) >= tft.width()) w = tft.width() - x;if ((y + h - 1) >= tft.height()) h = tft.height() - y;// Set TFT address window to clipped image boundstft.setAddrWindow(x, y, x + w - 1, y + h - 1);for (row = 0; row < h; row++) { // For each scanline...// Seek to start of scan line. It might seem labor-// intensive to be doing this on every line, but this// method covers a lot of gritty details like cropping// and scanline padding. Also, the seek only takes// place if the file position actually needs to change// (avoids a lot of cluster math in SD library).if (flip) // Bitmap is stored bottom-to-top order (normal BMP)pos = bmpImageoffset + (bmpHeight - 1 - row) * rowSize;else // Bitmap is stored top-to-bottompos = bmpImageoffset + row * rowSize;if (bmpFile.position() != pos) { // Need seek?bmpFile.seek(pos);buffidx = sizeof(sdbuffer); // Force buffer reload}for (col = 0; col < w; col++) { // For each column... // Time to read more pixel data? if (buffidx >= sizeof(sdbuffer)) { // Indeed// Push LCD buffer to the display firstif (lcdidx > 0) {tft.pushColors(lcdbuffer, lcdidx, first);lcdidx = 0;first = false;}bmpFile.read(sdbuffer, sizeof(sdbuffer));buffidx = 0; // Set index to beginning}// Convert pixel from BMP to TFT formatb = sdbuffer[buffidx++];g = sdbuffer[buffidx++];r = sdbuffer[buffidx++];lcdbuffer[lcdidx++] = tft.color565(r, g, b);} // end pixel} // end scanline// Write any remaining data to LCDif (lcdidx > 0) {tft.pushColors(lcdbuffer, lcdidx, first);}Serial.print(F("Loaded in "));Serial.print(millis() - startTime);Serial.println(" ms");} // end goodBmp}}bmpFile.close();if (!goodBmp) Serial.println(F("BMP format not recognized."));}// These read 16- and 32-bit types from the SD card file.// BMP data is stored little-endian, Arduino is little-endian too.// May need to reverse subscript order if porting elsewhere.uint16_t read16(File f) {uint16_t result;((uint8_t *)&result)[0] = f.read(); // LSB((uint8_t *)&result)[1] = f.read(); // MSBreturn result;}uint32_t read32(File f) {uint32_t result;((uint8_t *)&result)[0] = f.read(); // LSB((uint8_t *)&result)[1] = f.read();((uint8_t *)&result)[2] = f.read();((uint8_t *)&result)[3] = f.read(); // MSBreturn result;}
To display pictures on this LCD you should save the picture in 24bit BMP colored format and size of 240*320. Then move them to SD card and put the SD card in the LCD shield. we use the following function to display pictures. This function has 3 arguments; the first one stands for the pictures name, and the second and third arguments are for length and width coordinates of the top left corner of the picture.bmpdraw(“filename.bmp”,x,y);
Create A Paint App w/ Arduino 2.4 Touchscreen/*This code is TFTLCD Library Example*/#include #include #include #if defined(__SAM3X8E__)#undef __FlashStringHelper::F(string_literal)#define F(string_literal) string_literal#endif#define YP A3#define XM A2#define YM 9#define XP 8#define TS_MINX 150#define TS_MINY 120#define TS_MAXX 920#define TS_MAXY 940TouchScreen ts = TouchScreen(XP, YP, XM, YM, 300);#define LCD_CS A3#define LCD_CD A2#define LCD_WR A1#define LCD_RD A0#define LCD_RESET A4#define BLACK 0x0000#define BLUE 0x001F#define RED 0xF800#define GREEN 0x07E0#define CYAN 0x07FF#define MAGENTA 0xF81F#define YELLOW 0xFFE0#define WHITE 0xFFFFAdafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET);#define BOXSIZE 40#define PENRADIUS 3int oldcolor, currentcolor;void setup(void) {Serial.begin(9600);Serial.println(F("Paint!"));tft.reset();uint16_t identifier = tft.readID();if(identifier == 0x9325) {Serial.println(F("Found ILI9325 LCD driver"));} else if(identifier == 0x9328) {Serial.println(F("Found ILI9328 LCD driver"));} else if(identifier == 0x7575) {Serial.println(F("Found HX8347G LCD driver"));} else if(identifier == 0x9341) {Serial.println(F("Found ILI9341 LCD driver"));} else if(identifier == 0x8357) {Serial.println(F("Found HX8357D LCD driver"));} else {Serial.print(F("Unknown LCD driver chip: "));Serial.println(identifier, HEX);Serial.println(F("If using the Adafruit 2.4\" TFT Arduino shield, the line:"));Serial.println(F(" #define USE_ADAFRUIT_SHIELD_PINOUT"));Serial.println(F("should appear in the library header (Adafruit_TFT.h)."));Serial.println(F("If using the breakout board, it should NOT be #defined!"));Serial.println(F("Also if using the breakout, double-check that all wiring"));Serial.println(F("matches the tutorial."));return;}tft.begin(identifier);tft.fillScreen(BLACK);tft.fillRect(0, 0, BOXSIZE, BOXSIZE, RED);tft.fillRect(BOXSIZE, 0, BOXSIZE, BOXSIZE, YELLOW);tft.fillRect(BOXSIZE*2, 0, BOXSIZE, BOXSIZE, GREEN);tft.fillRect(BOXSIZE*3, 0, BOXSIZE, BOXSIZE, CYAN);tft.fillRect(BOXSIZE*4, 0, BOXSIZE, BOXSIZE, BLUE);tft.fillRect(BOXSIZE*5, 0, BOXSIZE, BOXSIZE, MAGENTA);tft.drawRect(0, 0, BOXSIZE, BOXSIZE, WHITE);currentcolor = RED;pinMode(13, OUTPUT);}#define MINPRESSURE 10#define MAXPRESSURE 1000void loop(){digitalWrite(13, HIGH);TSPoint p = ts.getPoint();digitalWrite(13, LOW);pinMode(XM, OUTPUT);pinMode(YP, OUTPUT);if (p.z > MINPRESSURE && p.z < MAXPRESSURE) {if (p.y < (TS_MINY-5)) {Serial.println("erase");tft.fillRect(0, BOXSIZE, tft.width(), tft.height()-BOXSIZE, BLACK);}p.x = map(p.x, TS_MINX, TS_MAXX, tft.width(), 0);p.y = map(p.y, TS_MINY, TS_MAXY, tft.height(), 0);if (p.y < BOXSIZE) {oldcolor = currentcolor;if (p.x < BOXSIZE) {currentcolor = RED;tft.drawRect(0, 0, BOXSIZE, BOXSIZE, WHITE);} else if (p.x < BOXSIZE*2) {currentcolor = YELLOW;tft.drawRect(BOXSIZE, 0, BOXSIZE, BOXSIZE, WHITE);} else if (p.x < BOXSIZE*3) {currentcolor = GREEN;tft.drawRect(BOXSIZE*2, 0, BOXSIZE, BOXSIZE, WHITE);} else if (p.x < BOXSIZE*4) {currentcolor = CYAN;tft.drawRect(BOXSIZE*3, 0, BOXSIZE, BOXSIZE, WHITE);} else if (p.x < BOXSIZE*5) {currentcolor = BLUE;tft.drawRect(BOXSIZE*4, 0, BOXSIZE, BOXSIZE, WHITE);} else if (p.x < BOXSIZE*6) { currentcolor = MAGENTA; tft.drawRect(BOXSIZE*5, 0, BOXSIZE, BOXSIZE, WHITE); } if (oldcolor != currentcolor) { if (oldcolor == RED) tft.fillRect(0, 0, BOXSIZE, BOXSIZE, RED); if (oldcolor == YELLOW) tft.fillRect(BOXSIZE, 0, BOXSIZE, BOXSIZE, YELLOW); if (oldcolor == GREEN) tft.fillRect(BOXSIZE*2, 0, BOXSIZE, BOXSIZE, GREEN); if (oldcolor == CYAN) tft.fillRect(BOXSIZE*3, 0, BOXSIZE, BOXSIZE, CYAN); if (oldcolor == BLUE) tft.fillRect(BOXSIZE*4, 0, BOXSIZE, BOXSIZE, BLUE); if (oldcolor == MAGENTA) tft.fillRect(BOXSIZE*5, 0, BOXSIZE, BOXSIZE, MAGENTA); } } if (((p.y-PENRADIUS) > BOXSIZE) && ((p.y+PENRADIUS) < tft.height())) {tft.fillCircle(p.x, p.y, PENRADIUS, currentcolor);}}}
Final NotesIf you want to display pictures without using an SD card, you can convert it to code and then display it. You can display even several photos sequentially without delay to create an animation. (Check this)But be aware that in this case, Arduino UNO may not be suitable (because of low processor speed). We recommend using the Arduino Mega or Arduino DUE.

The shield is fully assembled, tested, and ready to go. No wiring, no soldering! Simply plug it in and load up the library - you"ll have it running in under 10 minutes!
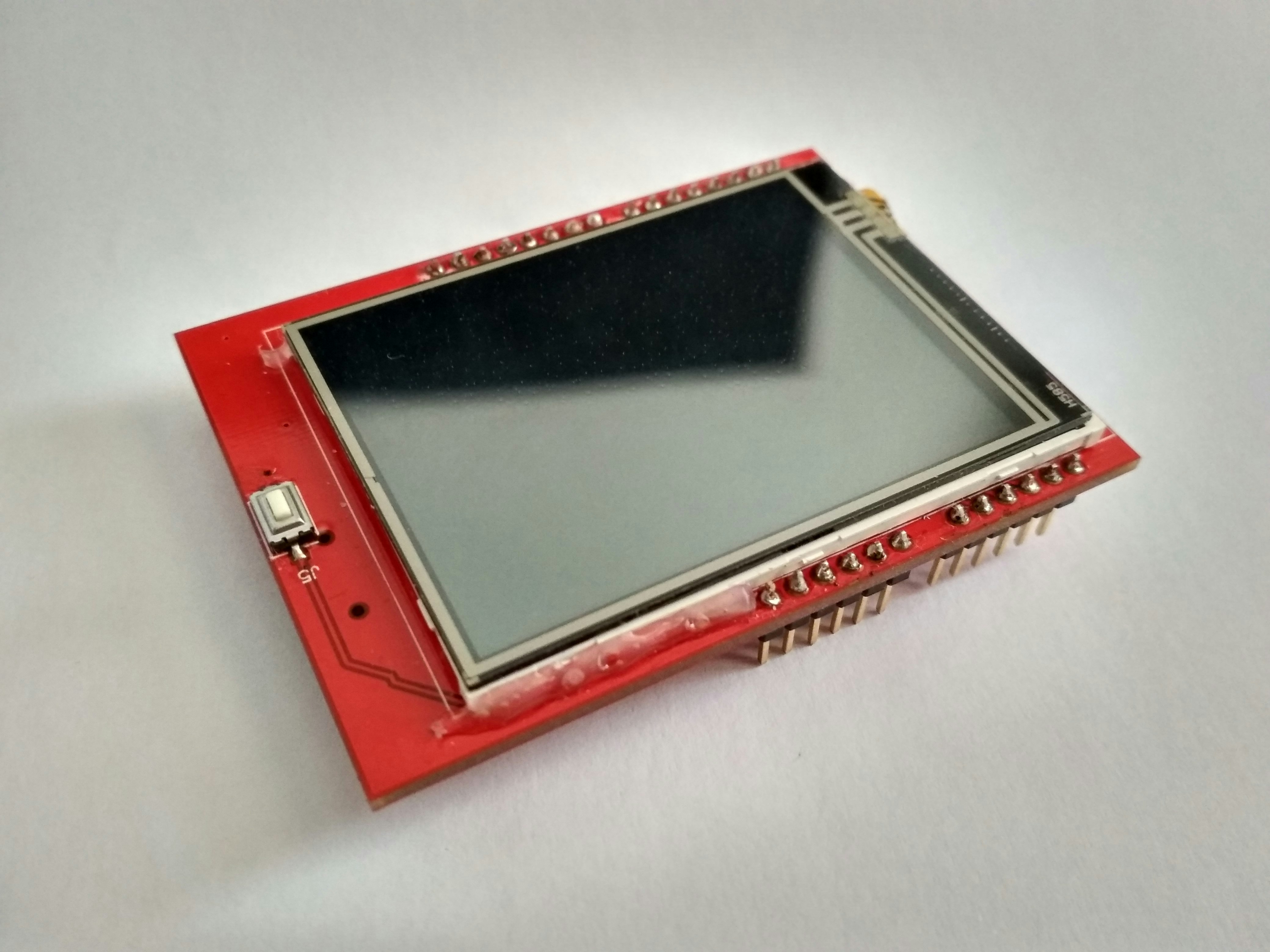
The shield is fully assembled, tested, and ready to go. No wiring, no soldering! Simply plug it in and load up the library - you"ll have it running in under 10 minutes!

(1) Due to the high risk of package lost recently, we have to stop using the free shipping way these days. Hope for your kind understanding! And the $5 is for tracking number and delivery insurance ^0^ Enjoy order from us.
2) Chinese Holiday Reminder: During annual Chinese holidays, services from certain suppliers and carriers may be affected, and delivery for orders placed around the following times may be delayed by 3 - 7 days: Chinese New Year; Chinese National Day,etc.
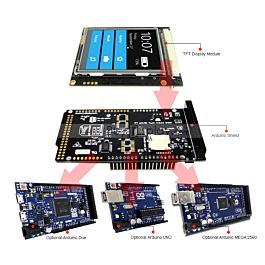
You may return most new, unopened items within 30 days of delivery for a full refund. We"ll also pay the return shipping costs if the return is a result of our error (you received an incorrect or defective item, etc.).
You should expect to receive your refund within four weeks of giving your package to the return shipper, however, in many cases you will receive a refund more quickly. This time period includes the transit time for us to receive your return from the shipper (5 to 10 business days), the time it takes us to process your return once we receive it (3 to 5 business days), and the time it takes your bank to process our refund request (5 to 10 business days).
When you place an order, we will estimate shipping and delivery dates for you based on the availability of your items and the shipping options you choose. Depending on the shipping provider you choose, shipping date estimates may appear on the shipping quotes page.
Please also note that the shipping rates for many items we sell are weight-based. The weight of any such item can be found on its detail page. To reflect the policies of the shipping companies we use, all weights will be rounded up to the next full pound.
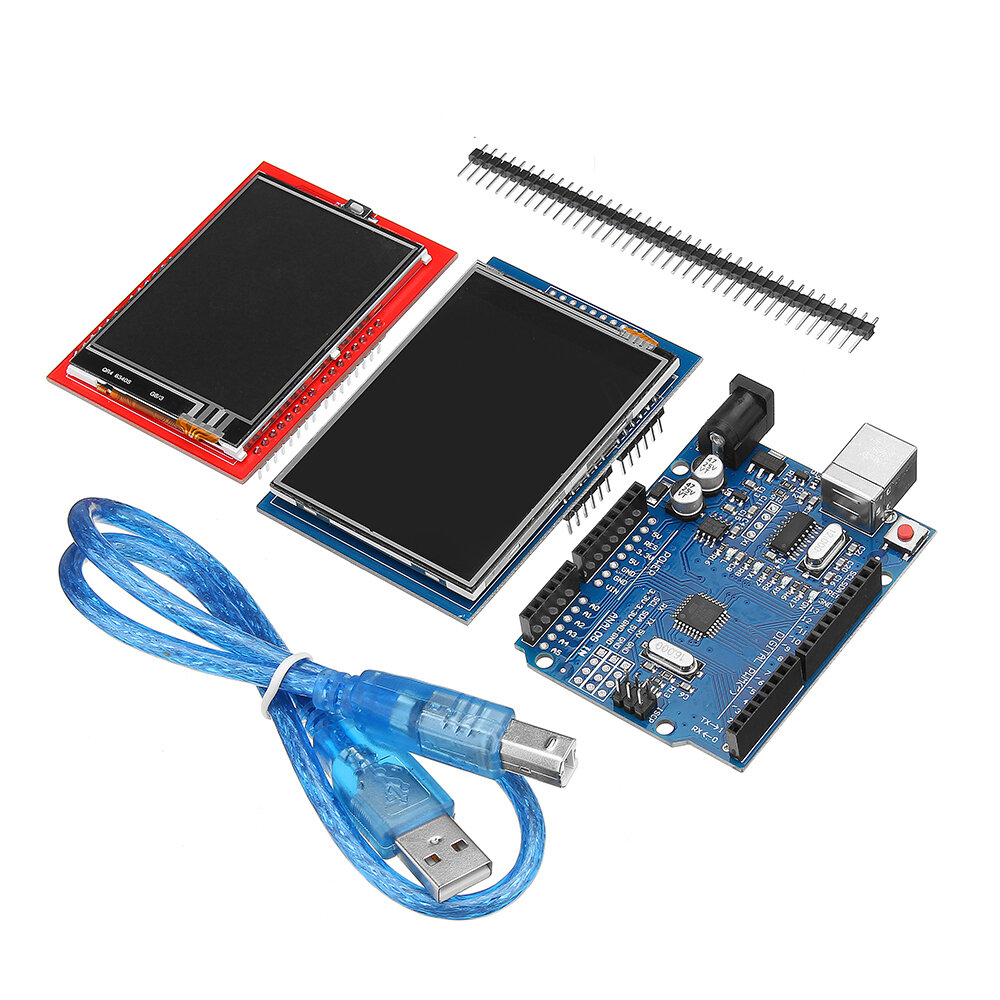
Spice up your Arduino project with a beautiful touchscreen display shield with built in microSD card connection. This TFT display is 2.4" diagonal and colorful (18-bit 262,000 different shades)! 240x320 pixels with individual pixel control. As a bonus, this display has a optional capacitive touch panel and resistive touch panel with controller XPT2046 attached by default.
The shield is fully assembled, tested and ready to go. No wiring, no soldering! Simply plug it in and load up our library - you"ll have it running in under 10 minutes! Works best with any classic Arduino (UNO/Due/Mega 2560).
This display shield has a controller built into it with RAM buffering, so that almost no work is done by the microcontroller. You can connect more sensors, buttons and LEDs.
Of course, we wouldn"t just leave you with a datasheet and a "good luck!" - we"ve written a full open source graphics library at the bottom of this page that can draw pixels, lines, rectangles, circles and text. We also have a touch screen library that detects x,y and z (pressure) and example code to demonstrate all of it. The code is written for Arduino but can be easily ported to your favorite microcontroller!
If you"ve had a lot of Arduino DUEs go through your hands (or if you are just unlucky), chances are you’ve come across at least one that does not start-up properly.The symptom is simple: you power up the Arduino but it doesn’t appear to “boot”. Your code simply doesn"t start running.You might have noticed that resetting the board (by pressing the reset button) causes the board to start-up normally.The fix is simple,here is the solution.
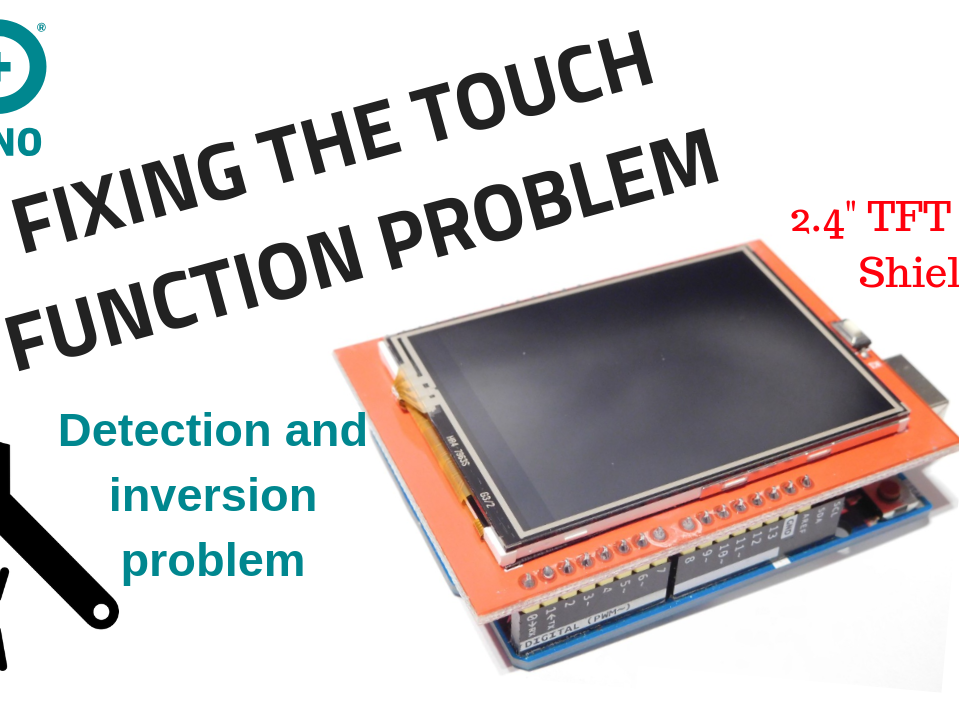
The 2.4 inch TFT LCD touch screen module suitable for Arduino, provides a large touchscreen display shield with a built in microSD socket offering a high resolution colourful display to your Arduino.

Even on ebay"s website it is mentioned that I can"t use 2.4" TFT LCD Shield display on attach to Arduino Mega. The problem is that I bought this shield by mistake. I want to put this shield onto Arduino Mega 2560. Is there a way to combine Mega and 2.4" Display Shield?

// For better pressure precision, we need to know the resistance // between X+ and X- Use any multimeter to read it // For the one we"re using, its 300 ohms across the X plate TouchScreen ts = TouchScreen(XP, YP, XM, YM, 300);
tft.fillRect(0, 0, BOXSIZE, BOXSIZE, RED); tft.fillRect(BOXSIZE, 0, BOXSIZE, BOXSIZE, YELLOW); tft.fillRect(BOXSIZE*2, 0, BOXSIZE, BOXSIZE, GREEN); tft.fillRect(BOXSIZE*3, 0, BOXSIZE, BOXSIZE, CYAN); tft.fillRect(BOXSIZE*4, 0, BOXSIZE, BOXSIZE, BLUE); tft.fillRect(BOXSIZE*5, 0, BOXSIZE, BOXSIZE, MAGENTA); // tft.fillRect(BOXSIZE*6, 0, BOXSIZE, BOXSIZE, WHITE); tft.drawRect(0, 0, BOXSIZE, BOXSIZE, WHITE); currentcolor = RED; pinMode(13, OUTPUT); }
void loop() { digitalWrite(13, HIGH); // Recently Point was renamed TSPoint in the TouchScreen library // If you are using an older version of the library, use the // commented definition instead. Point p = ts.getPoint(); // TSPoint p = ts.getPoint(); digitalWrite(13, LOW);
// if sharing pins, you"ll need to fix the directions of the touchscreen pins //pinMode(XP, OUTPUT); pinMode(XM, OUTPUT); pinMode(YP, OUTPUT); //pinMode(YM, OUTPUT);
if (p.z > MINPRESSURE && p.z < MAXPRESSURE) { /* Serial.print("X = "); Serial.print(p.x); Serial.print("\tY = "); Serial.print(p.y); Serial.print("\tPressure = "); Serial.println(p.z); */ if (p.y < (TS_MINY-5)) { Serial.println("erase"); // press the bottom of the screen to erase tft.fillRect(0, BOXSIZE, tft.width(), tft.height()-BOXSIZE, BLACK); } // scale from 0->1023 to tft.width p.x = tft.width()-(map(p.x, TS_MINX, TS_MAXX, tft.width(), 0)); p.y = tft.height()-(map(p.y, TS_MINY, TS_MAXY, tft.height(), 0)); /* Serial.print("("); Serial.print(p.x); Serial.print(", "); Serial.print(p.y); Serial.println(")"); */ if (p.y < BOXSIZE) { oldcolor = currentcolor;
if (p.x < BOXSIZE) { currentcolor = RED; tft.drawRect(0, 0, BOXSIZE, BOXSIZE, WHITE); } else if (p.x < BOXSIZE*2) { currentcolor = YELLOW; tft.drawRect(BOXSIZE, 0, BOXSIZE, BOXSIZE, WHITE); } else if (p.x < BOXSIZE*3) { currentcolor = GREEN; tft.drawRect(BOXSIZE*2, 0, BOXSIZE, BOXSIZE, WHITE); } else if (p.x < BOXSIZE*4) { currentcolor = CYAN; tft.drawRect(BOXSIZE*3, 0, BOXSIZE, BOXSIZE, WHITE); } else if (p.x < BOXSIZE*5) { currentcolor = BLUE; tft.drawRect(BOXSIZE*4, 0, BOXSIZE, BOXSIZE, WHITE); } else if (p.x < BOXSIZE*6) { currentcolor = MAGENTA; tft.drawRect(BOXSIZE*5, 0, BOXSIZE, BOXSIZE, WHITE); }
if (oldcolor != currentcolor) { if (oldcolor == RED) tft.fillRect(0, 0, BOXSIZE, BOXSIZE, RED); if (oldcolor == YELLOW) tft.fillRect(BOXSIZE, 0, BOXSIZE, BOXSIZE, YELLOW); if (oldcolor == GREEN) tft.fillRect(BOXSIZE*2, 0, BOXSIZE, BOXSIZE, GREEN); if (oldcolor == CYAN) tft.fillRect(BOXSIZE*3, 0, BOXSIZE, BOXSIZE, CYAN); if (oldcolor == BLUE) tft.fillRect(BOXSIZE*4, 0, BOXSIZE, BOXSIZE, BLUE); if (oldcolor == MAGENTA) tft.fillRect(BOXSIZE*5, 0, BOXSIZE, BOXSIZE, MAGENTA); } } if (((p.y-PENRADIUS) > BOXSIZE) && ((p.y+PENRADIUS) < tft.height())) { tft.fillCircle(p.x, p.y, PENRADIUS, currentcolor); } } }
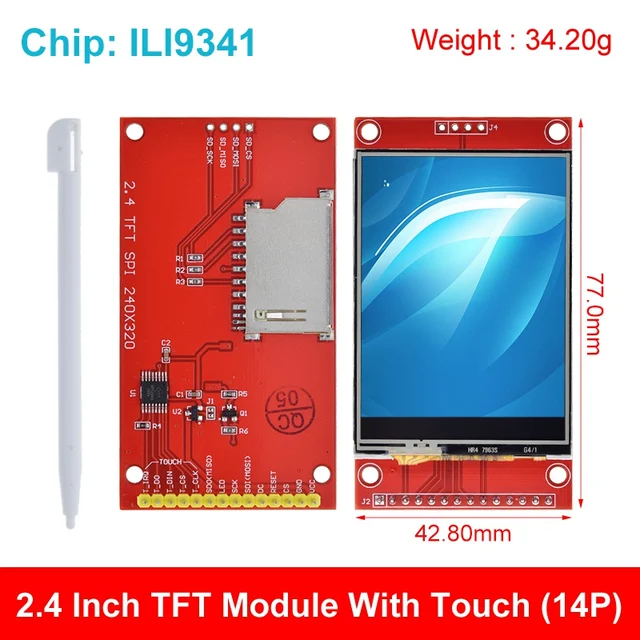
Andhra Pradesh, Arunachal Pradesh, Assam, Bihar, Chhattisgarh, Goa, Gujarat, Haryana, Himachal Pradesh, Jammu and Kashmir, Jharkhand, Karnataka, Kerala, Madhya Pradesh, Maharashtra, Manipur, Meghalaya, Mizoram, Nagaland, Orissa, Punjab, Rajasthan, Sikkim, Tamil Nadu, Telangana, Tripura, Uttaranchal, Uttar Pradesh, West Bengal and major cities including Ahmednagar, Aizawl, Ajmer, Akola, Alappuzha, Aligarh, Allahabad, Alleppey, Almora, Alwar, Amarnath, Ambattur, Ambernath, Amravati, Amritsar, Anantagir, Anantapur, Andaman, Araku, Arrah, Asansol, Aurangabad, Avadi, Ayodhya, Ayurveda, Badrinath, Bally, Bandhavgarh, Bangalore, Baranagar, Barasat, Bardhaman, Bareilly, Baroda, Bastar, Bathinda, Beach, Beaches, Begusarai, Belgaum, Bellary, Bhagalpur, Bharatpur, Bhatpara, Bhavnagar, Bhilai, Bhilwara, Bhimtal, Bhiwandi, Bhopal, Bhubaneswar, Bhuj, Bidar, Bihar, Bijapur, Bikaner, Bilaspur, Bird, Bodh, Bokaro, Brahmapur, Bulandshahr, Calicut, Chail, Chamba, Chandigarh, Chandrapur, Chennai, Chennai, Cherai, Cherrapunji, Chidambaram, Chikhaldara, Chopta, Coimbatore, Coimbatore, Coonoor, Coorg, Corbett, Cotigao, Cuttack, Dadra, Dalhousie, Daman, Darbhanga, Darjeeling, Davanagere, Dehradun, Delhi, Devi, Devikulam, Dewas, Dhanaulti, Dhanbad, Dharamashala, Dhule, Dindigul, Diu, Dudhwa, Durg, Durgapur, Dwaraka, Etawah, Faridabad, Farrukhabad, Firozabad, Flowers, Gandhidham, Gandhinagar, Gangotri, Gangtok, Gaya, Ghaziabad, Gir, Goa, Gopalpur, Gorakhpur, Great, Gulbarga, Gulmarg, Guntur, Gurgaon, Guruvayoor, Guwahati, Gwalior, Hampi, Hapur, Haridwar, Haveli, Hills, Himalayan, Hisar, Hogenakkal, Horsley-Hills, Howrah, Hubballi-Dharwad, Hyderabad, Hyderabad, Ichalkaranji, Idukki, Imphal, Indore, Islands, Itangar, Jabalpur, Jaipur, Jaisalmer, Jalandhar, Jalgaon, Jalna, Jammu, Jamnagar, Jamshedpur, Jhansi, Jodhpur, Junagadh, Kadapa, Kakinada, Kalyan-Dombivali, Kamarhati, Kanchipuram, Kanha, Kanpur, Kanyakumari, Karawal Nagar, Kargil, Karimnagar, Karnal, Karwar, Katihar, Kausani, Kedarnath, Keoladeoghana, Khajuraho, Khammam, Kirari Suleman Nagar, Kochi, Kodaikanal, Kolhapur, Kolkata, Kollam, Konark, Korba, Kota, Kotagiri, Kottakkal, Kovalam, Kozhikode, Kudremukh, Kullu, Kulti, Kumaon, Kumarakom, Kurnool, Kurukshetra, Lakshadweep, Latur, Life, Loni, Madurai, Malpe, Manas, Mango, Maravanthe, Margoa, Mau, Meerut, Mira-Bhayandar, Mirzapur, Moradabad, Mount, Mumbai, Mussoorie, Muzaffarpur, Nalanda, Nanda, Nanded, Nandi-Hills, Nashik, National, Navi Mumbai, Nellore, Netravali, Nizamabad, Noida, North Dumdum, Orchha, Ozhukarai, Pali, Panipat, Parbhani, Patiala, Patnitop, Pattadakkal, Pondicherry, Puducherry, Pune, Puri, Purnia, Pushkar, Rajahmundry, Rajgir, Rameshwaram, Rampur, Ranchi, Ranikhet, Ranthambore, Rohtak, Rourkela, Sagar, Sangli-Miraj & Kupwad, Sariska, Satna, Shillong, Sikar, Solapur, South Dumdum, Sri Ganganagar, Srinagar, Surat, Tezpur, Thane, Thanjavur, Thiruvananthapuram, Thoothukudi, Thrissur, Tiruchirappalli, Tirunelveli, Tirupati, Tirupur, Tumkur, Udaipur, Ujjain, Vaishali, Vasai-Virar, Visakhapatnam, Vishakhapatnam, Vizianagaram, Warangal, Warangal, Wayanad, Yercaud, Zanskar, Nagpur etc. You may buy latest electronics components, accessories and development boards from us at affordable prices. Buy Raspberry Pi, Arduino, AVR, ARM, 8051 Development Boards, Wireless and Wired, Microcontroller and ICS, Robotics & Accessories, Prototyping Components, Educational Kits etc. We also provide technical assistance for products purchased from our store and supplement it with schematics, code examples and demonstrations.