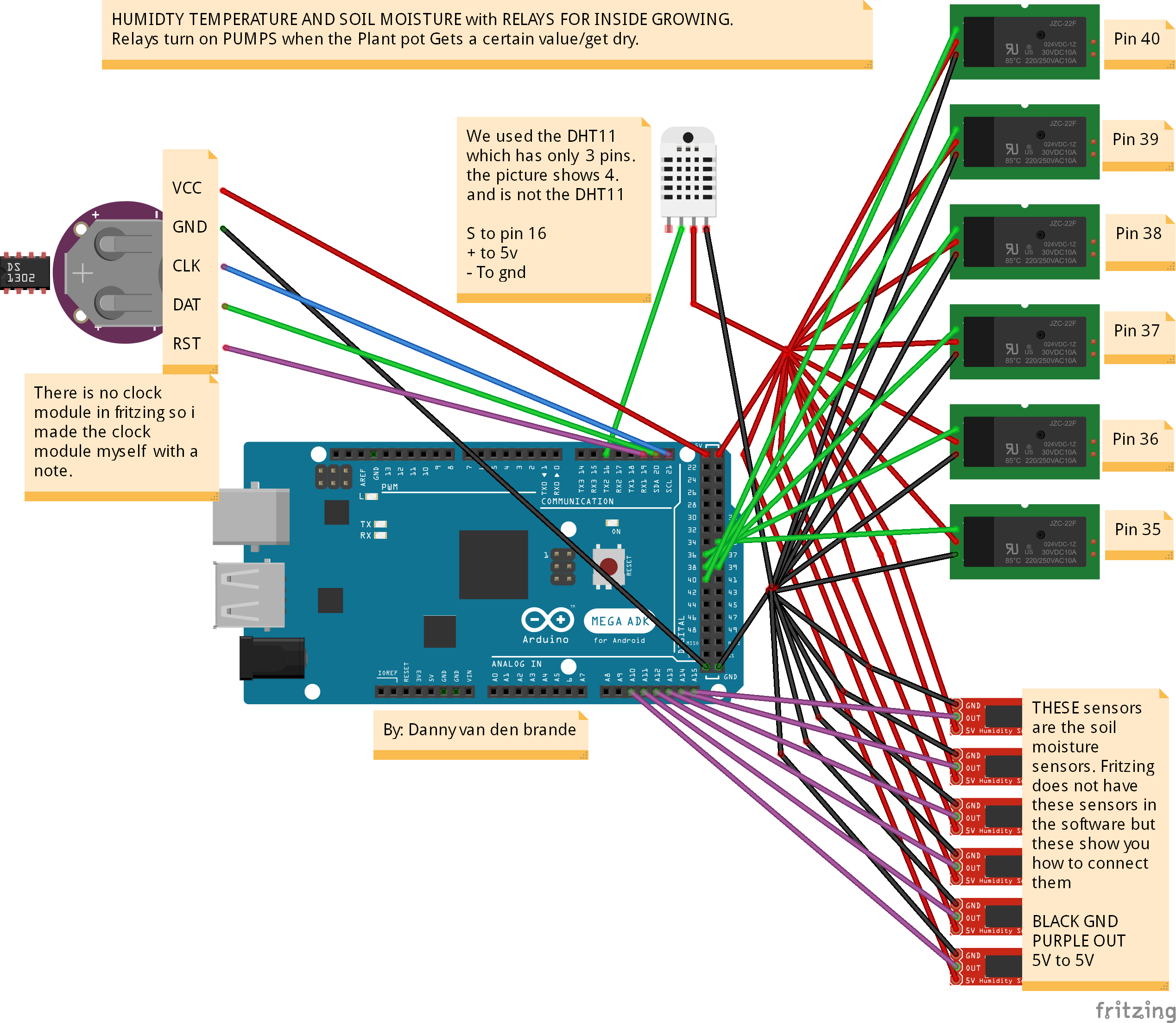
I changed the Adafruit libraries for TFT: GFX , TFTLCD and TouchScreen. I join all in this one library, the library SPFD5408, to avoid problems with duplicate libraries and enables also have the original library Adafruit ready for use in other projects with another TFT hardware.

//Author Danny van den brande.#include "DHT.h"#include // Core graphics library#include // Hardware-specific library#include // BEGIN CLOCK#include //clock module DS1302#include //Need for clock module#define DS1302_SCLK_PIN 21// Arduino pin for the Serial Clock//PIN 21 (SCLK_PIN) = CLK on CLOCK and SCL on arduino#define DS1302_IO_PIN 20// Arduino pin for the Data I/O//PIN 20 (IO_PIN) = DAT on CLOCK and SDA on arduino#define DS1302_CE_PIN 19// Arduino pin for the Chip Enable//PIN 19 (CE_PIN) = RST on CLOCK and TX1 on arduino for this you can define any free Digital pin.#define bcd2bin(h,l) (((h)*10) + (l))#define bin2bcd_h(x) ((x)/10)#define bin2bcd_l(x) ((x)%10)#define DS1302_SECONDS 0x80#define DS1302_MINUTES 0x82#define DS1302_HOURS 0x84#define DS1302_DATE 0x86#define DS1302_MONTH 0x88#define DS1302_DAY 0x8A#define DS1302_YEAR 0x8C#define DS1302_ENABLE 0x8E#define DS1302_TRICKLE 0x90#define DS1302_CLOCK_BURST 0xBE#define DS1302_CLOCK_BURST_WRITE 0xBE#define DS1302_CLOCK_BURST_READ 0xBF#define DS1302_RAMSTART 0xC0#define DS1302_RAMEND 0xFC#define DS1302_RAM_BURST 0xFE#define DS1302_RAM_BURST_WRITE 0xFE#define DS1302_RAM_BURST_READ 0xFF#define DS1302_D0 0#define DS1302_D1 1#define DS1302_D2 2#define DS1302_D3 3#define DS1302_D4 4#define DS1302_D5 5#define DS1302_D6 6#define DS1302_D7 7#define DS1302_READBIT DS1302_D0// READBIT=1: read instruction// Bit for clock (0) or ram (1) area,// called R/C-bit (bit in address)#define DS1302_RC DS1302_D6// Seconds Register#define DS1302_CH DS1302_D7// 1 = Clock Halt, 0 = start// Hour Register#define DS1302_AM_PM DS1302_D5// 0 = AM, 1 = PM#define DS1302_12_24 DS1302_D7// 0 = 24 hour, 1 = 12 hour// Enable Register#define DS1302_WP DS1302_D7// 1 = Write Protect, 0 = enabled#define DS1302_ROUT0 DS1302_D0#define DS1302_ROUT1 DS1302_D1#define DS1302_DS0 DS1302_D2#define DS1302_DS1 DS1302_D2#define DS1302_TCS0 DS1302_D4#define DS1302_TCS1 DS1302_D5#define DS1302_TCS2 DS1302_D6#define DS1302_TCS3 DS1302_D7// Bit for reading (bit in address)#define DS1302_READBIT DS1302_D0// READBIT=1: read instruction#define DHTPIN 16// what pin we"re connected to#define DHTTYPE DHT11// DHT 11#define DS1302_READBIT DS1302_D0// READBIT=1: read instruction#define LCD_CS A3// Chip Select goes to Analog 3#define LCD_CD A2// Command/Data goes to Analog 2#define LCD_WR A1// LCD Write goes to Analog 1#define LCD_RD A0// LCD Read goes to Analog 0#define LCD_RESET A4// Can alternately just connect to Arduino"s reset pin// Assign human-readable names to some common 16-bit color values:#define BLACK 0x0000#define BLUE 0x001F#define RED 0xF800#define GREEN 0x07E0#define CYAN 0x07FF#define MAGENTA 0xF81F#define YELLOW 0xFFE0#define WHITE 0xFFFF// OBJECTS LCD ET DHTAdafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET);DHT dht(DHTPIN, DHTTYPE);float hprev, tprev, hicprev;int moisture = 0;int moisture1 = 0;int moisture2 = 0;int moisture3 = 0;int moisture4 = 0;int moisture5 = 0;int sensorValue1;int sensorValue2;int sensorValue3;int sensorValue4;int sensorValue5;int sensorValue;int relay = 35;int relay1 = 36;int relay2 = 37;int relay3 = 38;int relay4 = 39;int relay5 = 40;// Structure for the first 8 registers.// These 8 bytes can be read at once with// the "clock burst" command.// Note that this structure contains an anonymous union.// It might cause a problem on other compilers.typedef struct ds1302_struct{uint8_t Seconds:4; // low decimal digit 0-9uint8_t Seconds10:3; // high decimal digit 0-5uint8_t CH:1; // CH = Clock Haltuint8_t Minutes:4;uint8_t Minutes10:3;uint8_t reserved1:1;union{struct{uint8_t Hour:4;uint8_t Hour10:2;uint8_t reserved2:1;uint8_t hour_12_24:1; // 0 for 24 hour format} h24;struct{uint8_t Hour:4;uint8_t Hour01:1;uint8_t AM_PM:1; // 0 for AM, 1 for PMuint8_t reserved2:1;uint8_t hour_12_24:1; // 1 for 12 hour format} h12;};uint8_t Date:4; // Day of month, 1 = first dayuint8_t Date10:2;uint8_t reserved3:2;uint8_t Month:4; // Month, 1 = Januaryuint8_t Month10:1;uint8_t reserved4:3;uint8_t Day:3; // Day of week, 1 = first day (any day)uint8_t reserved5:5;uint8_t Year:4; // Year, 0 = year 2000uint8_t Year10:4;uint8_t reserved6:7;uint8_t WP:1; // WP = Write Protect};void setup(){ds1302_struct rtc;Serial.begin(9600);// Serial.println(F("BLUECORE TECH"));pinMode (relay, OUTPUT);pinMode (relay1, OUTPUT);pinMode (relay2, OUTPUT);pinMode (relay3, OUTPUT);pinMode (relay4, OUTPUT);pinMode (relay5, OUTPUT);// digitalWrite (relay, HIGH);#ifdef USE_ADAFRUIT_SHIELD_PINOUT// Serial.println(F("Using Adafruit 2.8\" TFT Arduino Shield Pinout"));#else// Serial.println(F("Using Adafruit 2.8\" TFT Breakout Board Pinout"));#endif// Serial.print("TFT size is "); Serial.print(tft.width()); Serial.print("x"); Serial.println(tft.height());//tft.reset();uint16_t identifier = tft.readID();if(identifier == 0x9325) {// Serial.println(F("Found ILI9325 LCD driver"));} else if(identifier == 0x9327) {// Serial.println(F("Found ILI9327 LCD driver"));} else if(identifier == 0x9328) {// Serial.println(F("Found ILI9328 LCD driver"));} else if(identifier == 0x7575) {// Serial.println(F("Found HX8347G LCD driver"));} else if(identifier == 0x9341) {// Serial.println(F("Found ILI9341 LCD driver"));} else if(identifier == 0x8357) {// Serial.println(F("Found HX8357D LCD driver"));} else if(identifier == 0x0154) {// Serial.println(F("Found S6D0154 LCD driver"));}tft.begin(identifier);iniText();dht.begin();//CLOCK MODULE START// Start by clearing the Write Protect bit// Otherwise the clock data cannot be written// The whole register is written,// but the WP-bit is the only bit in that register.DS1302_write (DS1302_ENABLE, 0);// Disable Trickle Charger.DS1302_write (DS1302_TRICKLE, 0x00);// Remove the next define,// after the right date and time are set.// #define SET_DATE_TIME_JUST_ONCE //= 300)digitalWrite (relay, HIGH); //TURN ON/OFF OFF RELAY 0 for watering pumpelsedigitalWrite (relay, LOW);//SENSOR 1 set your sensor here!}// OPTIONAL SENSORS PLACES FOR VALUES ON SCREEN BEGINif (sensorValue) {tft.setTextSize(2);tft.setCursor(98, 127);tft.setTextColor(GREEN);tft.fillRect(93,117,67,34,BLACK);tft.println (moisture1/1.01);if (sensorValue1 >= 300)digitalWrite (relay1, HIGH); //TURN ON/OFF OFF RELAY 1 for watering pumpelsedigitalWrite (relay1, LOW);//SENSOR 2 set your sensor here!}if (sensorValue) {tft.setTextSize(2);tft.setCursor(98, 164);tft.setTextColor(GREEN);tft.fillRect(93,154,67,34,BLACK);tft.println (moisture2/1.01);if (sensorValue2 >= 300)digitalWrite (relay2, HIGH); //TURN ON/OFF OFF RELAY 2 for watering pumpelsedigitalWrite (relay2, LOW);//SENSOR 3 set your sensor here!} // BEGIN SECTION 2 - right sectionif (sensorValue) {tft.setCursor(10,60);tft.setTextSize(2);//tft.println ("BODEM VOCHT%");tft.setCursor(256, 90);tft.setTextColor(GREEN);tft.fillRect(250,80,67,34,BLACK);tft.println (moisture3/1.01);if (sensorValue3 >= 300)digitalWrite (relay3, HIGH); //TURN ON/OFF OFF RELAY 3 for watering pumpelsedigitalWrite (relay3, LOW);//SENSOR 4 set your sensor here!}if (sensorValue) {tft.setCursor(10,60);tft.setTextSize(2);//tft.println ("BODEM VOCHT%");tft.setCursor(256, 127);tft.setTextColor(GREEN);tft.fillRect(250,117,67,34,BLACK);tft.println (moisture4/1.01);if (sensorValue4 >= 300)digitalWrite (relay4, HIGH); //TURN ON/OFF OFF RELAY 4 for watering pumpelsedigitalWrite (relay4, LOW);//SENSOR 5 set your sensor here!}if (sensorValue) {tft.setCursor(10,60);tft.setTextSize(2);//tft.println ("BODEM VOCHT%");tft.setCursor(256, 164);tft.setTextColor(GREEN);tft.fillRect(250,154,67,34,BLACK);tft.println (moisture5/1.01);if (sensorValue5 >= 300)digitalWrite (relay5, HIGH); //TURN ON/OFF OFF RELAY 5 for watering pumpelsedigitalWrite (relay5, LOW);//SENSOR 6 set your sensor here!}// OPTIONAL SENSORS PLACES ON SCREEN ENDif (hprev != h) {tft.setCursor(10, 25);tft.setTextSize(3);tft.setTextColor(CYAN);tft.fillRect(3,25,103,25,BLACK);tft.print(h);hprev = h;}if (tprev != t) {tft.setCursor(118, 25);tft.setTextSize(3);tft.setTextColor(RED);tft.fillRect(111,25,101,25,BLACK);tft.print(t);tprev = t;}if (hicprev != hic) {tft.setCursor(225, 25);tft.setTextSize(3);tft.setTextColor(YELLOW);tft.fillRect(217,25,100,25,BLACK);tft.print(hic);hicprev = hic;}ds1302_struct rtc;char buffer[80]; // the code uses 70 characters.// Read all clock data at once (burst mode).DS1302_clock_burst_read( (uint8_t *) &rtc);//+++++++++++ BEGIN TEXT CLOCK TEXT+++++++++++tft.setTextSize(2);tft.setTextColor(GREEN);tft.setCursor(13, 220);tft.fillRect(3,215,115,25,BLACK);sprintf( buffer, "%02d:%02d:%02d ", \bcd2bin( rtc.h24.Hour10, rtc.h24.Hour), \bcd2bin( rtc.Minutes10, rtc.Minutes), \bcd2bin( rtc.Seconds10, rtc.Seconds));tft.print(buffer);tft.setTextSize(1);tft.setTextColor(BLACK);tft.setCursor(127, 218);tft.fillRect(122,215,195,25,GREEN);sprintf(buffer, "%d,%d," \"Dag %d van week,%d", \bcd2bin( rtc.Date10, rtc.Date), \bcd2bin( rtc.Month10, rtc.Month), \rtc.Day, \2000 + bcd2bin( rtc.Year10, rtc.Year));tft.println( buffer);tft.setTextSize(1);tft.setTextColor(BLACK);tft.setCursor(127, 230);// tft.fillRect(122,215,194,25,GREEN);sprintf(buffer, "%d,%d," \"Day %d of week,%d", \bcd2bin( rtc.Month10, rtc.Month), \bcd2bin( rtc.Date10, rtc.Date), \rtc.Day, \2000 + bcd2bin( rtc.Year10, rtc.Year));tft.println( buffer);//+++++++++++ EINDE CLOCK +++++++++++if(timeStatus() != timeSet) {tft.setTextSize(1.5);tft.setTextColor(BLACK);tft.setCursor(28, 198);///ERROR TEXT need to be coded correctlytft.fillRect(3,191,157,19,RED);//tft.print(F("CLOCK ERROR: SYNC!"));// return micros() - start;delay(1000);}}unsigned long iniText() {// unsigned long start = micros();tft.fillScreen(BLACK);tft.setRotation(3);tft.setTextSize(1);tft.setTextColor(WHITE);tft.setCursor(15,5);tft.println("Humidity %");tft.setCursor(119,10);tft.println("Temperature oC");tft.setCursor(235,5);tft.println("Heat Index"); //Gevoels temperatuurtft.setCursor(122,2);tft.println ("BlueCore TECH"); //Put your NAME here COMPANY NAMEtft.setCursor(190,198);tft.println ("ArduinoSensors.NL"); //Put your NAME here COMPANY NAME website//TEXT SENSORStft.setCursor(10,90);tft.setTextColor(WHITE);tft.println ("SENSOR:1");tft.setCursor(10,127);tft.println ("SENSOR:2");tft.setCursor(10,164);tft.println ("SENSOR:3");tft.setCursor(170,90);tft.println ("SENSOR:4");tft.setCursor(170,127);tft.println ("SENSOR:5");tft.setCursor(170,164);tft.println ("SENSOR:6");// end TEXT SENSORS//Interface DESIGN BEGINtft.fillRect(0,0,345,1,WHITE); //Top line header whitetft.fillRect(0,19,345,2,WHITE); //Top line header 2 whitetft.fillRect(0,20,345,5,BLACK); //Top line header blacktft.fillRect(106,0,5,50,WHITE); //center vertical line header lefttft.fillRect(212,0,5,50,WHITE); //center vertical line header righttft.fillRect(0,50,345,5,WHITE); //bottom line header.tft.fillRect(160,78,5,135,WHITE);//center vertical linetft.fillRect(317,0,5,240,WHITE);//center vertical line righttft.fillRect(0,0,3,240,WHITE);//center vertical line lefttft.fillRect(0,210,345,5,WHITE);//BOTTOM LINE Footertft.fillRect(118,215,4,25,WHITE);//BOTTOM LINE Footer2 vertical smalltft.fillRect(0,210,345,5,WHITE);//BOTTOM LINE Footertft.fillRect(0,78,345,2,WHITE);//top line center screentft.fillRect(0,115,345,2,WHITE);//line 2 center screentft.fillRect(0,152,345,2,WHITE);//line 3 center screentft.fillRect(0,189,345,2,WHITE);//line 4 center screen//Interface DESIGN END// return micros() - start;}void DS1302_clock_burst_read( uint8_t *p)///BEGIN CLOCK MODULE PART2{int i;_DS1302_start();// Instead of the address,// the CLOCK_BURST_READ command is issued// the I/O-line is released for the data_DS1302_togglewrite( DS1302_CLOCK_BURST_READ, true);for( i=0; i<8; i++){*p++ = _DS1302_toggleread();}_DS1302_stop();}// --------------------------------------------------------// DS1302_clock_burst_write//// This function writes 8 bytes clock data in burst mode// to the DS1302.//// This function may be called as the first function,// also the pinMode is set.//void DS1302_clock_burst_write( uint8_t *p){int i;_DS1302_start();// Instead of the address,// the CLOCK_BURST_WRITE command is issued.// the I/O-line is not released_DS1302_togglewrite( DS1302_CLOCK_BURST_WRITE, false);for( i=0; i<8; i++){// the I/O-line is not released_DS1302_togglewrite( *p++, false);}_DS1302_stop();}// --------------------------------------------------------// DS1302_read//// This function reads a byte from the DS1302// (clock or ram).//// The address could be like "0x80" or "0x81",// the lowest bit is set anyway.//// This function may be called as the first function,// also the pinMode is set.//uint8_t DS1302_read(int address){uint8_t data;// set lowest bit (read bit) in addressbitSet( address, DS1302_READBIT);_DS1302_start();// the I/O-line is released for the data_DS1302_togglewrite( address, true);data = _DS1302_toggleread();_DS1302_stop();return (data);}// --------------------------------------------------------// DS1302_write//// This function writes a byte to the DS1302 (clock or ram).//// The address could be like "0x80" or "0x81",// the lowest bit is cleared anyway.//// This function may be called as the first function,// also the pinMode is set.//void DS1302_write( int address, uint8_t data){// clear lowest bit (read bit) in addressbitClear( address, DS1302_READBIT);_DS1302_start();// don"t release the I/O-line_DS1302_togglewrite( address, false);// don"t release the I/O-line_DS1302_togglewrite( data, false);_DS1302_stop();}// --------------------------------------------------------// _DS1302_start//// A helper function to setup the start condition.//// An "init" function is not used.// But now the pinMode is set every time.// That"s not a big deal, and it"s valid.// At startup, the pins of the Arduino are high impedance.// Since the DS1302 has pull-down resistors,// the signals are low (inactive) until the DS1302 is used.void _DS1302_start( void){digitalWrite( DS1302_CE_PIN, LOW); // default, not enabledpinMode( DS1302_CE_PIN, OUTPUT);digitalWrite( DS1302_SCLK_PIN, LOW); // default, clock lowpinMode( DS1302_SCLK_PIN, OUTPUT);pinMode( DS1302_IO_PIN, OUTPUT);digitalWrite( DS1302_CE_PIN, HIGH); // start the sessiondelayMicroseconds( 4); // tCC = 4us}// --------------------------------------------------------// _DS1302_stop//// A helper function to finish the communication.//void _DS1302_stop(void){// Set CE lowdigitalWrite( DS1302_CE_PIN, LOW);delayMicroseconds( 4); // tCWH = 4us}// --------------------------------------------------------// _DS1302_toggleread//// A helper function for reading a byte with bit toggle//// This function assumes that the SCLK is still high.//uint8_t _DS1302_toggleread( void){uint8_t i, data;data = 0;for( i = 0; i <= 7; i++){// Issue a clock pulse for the next databit.// If the "togglewrite" function was used before// this function, the SCLK is already high.digitalWrite( DS1302_SCLK_PIN, HIGH);delayMicroseconds( 1);// Clock down, data is ready after some time.digitalWrite( DS1302_SCLK_PIN, LOW);delayMicroseconds( 1); // tCL=1000ns, tCDD=800ns// read bit, and set it in place in "data" variablebitWrite( data, i, digitalRead( DS1302_IO_PIN));}return( data);}// --------------------------------------------------------// _DS1302_togglewrite//// A helper function for writing a byte with bit toggle//// The "release" parameter is for a read after this write.// It will release the I/O-line and will keep the SCLK high.//void _DS1302_togglewrite( uint8_t data, uint8_t release){int i;for( i = 0; i <= 7; i++){// set a bit of the data on the I/O-linedigitalWrite( DS1302_IO_PIN, bitRead(data, i));delayMicroseconds( 1); // tDC = 200ns// clock up, data is read by DS1302digitalWrite( DS1302_SCLK_PIN, HIGH);delayMicroseconds( 1); // tCH = 1000ns, tCDH = 800nsif( release && i == 7){// If this write is followed by a read,// the I/O-line should be released after// the last bit, before the clock line is made low.// This is according the datasheet.// I have seen other programs that don"t release// the I/O-line at this moment,// and that could cause a shortcut spike// on the I/O-line.pinMode( DS1302_IO_PIN, INPUT);// For Arduino 1.0.3, removing the pull-up is no longer needed.// Setting the pin as "INPUT" will already remove the pull-up.// digitalWrite (DS1302_IO, LOW); // remove any pull-up}else{digitalWrite( DS1302_SCLK_PIN, LOW);delayMicroseconds( 1); // tCL=1000ns, tCDD=800ns}}///////////////////////////// END CLOCK MODULE part 2}
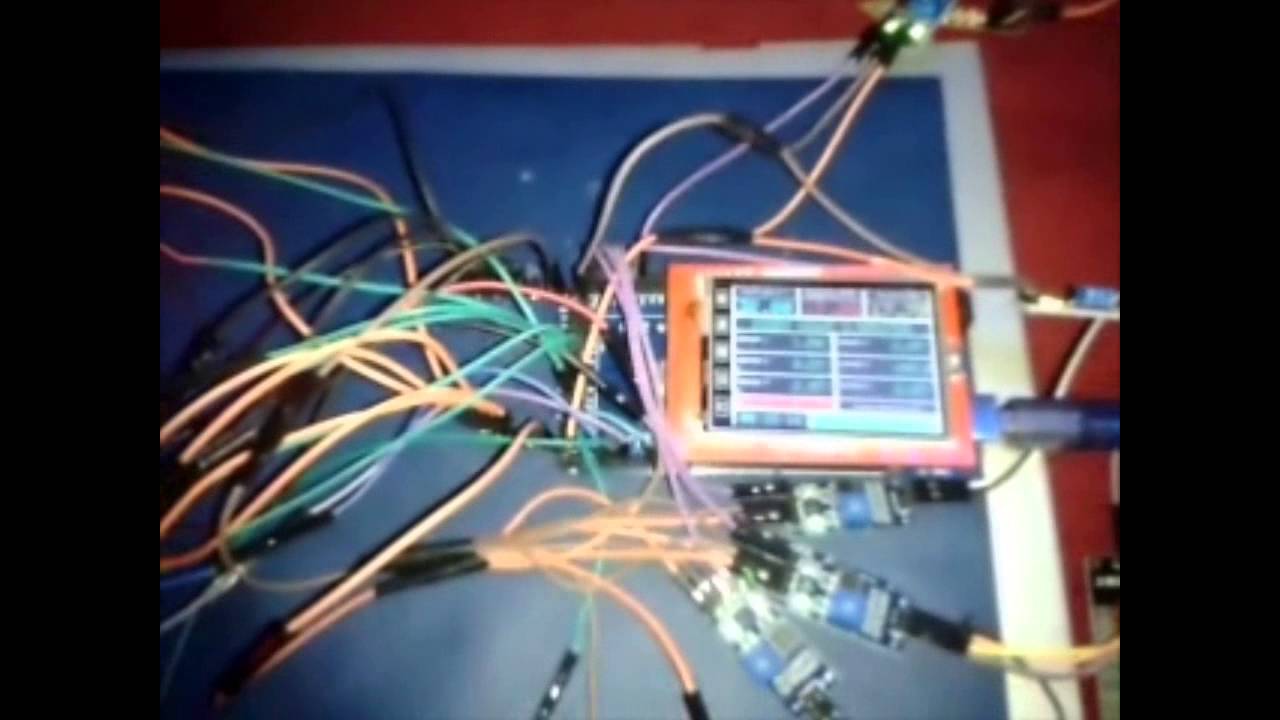
For questions about connecting a Crystalfontz TFT, what type of LCD is the best choice for your application, or any other questions (technical or availability), please contact our knowledgeable and friendly support staff via email, phone, or chat.
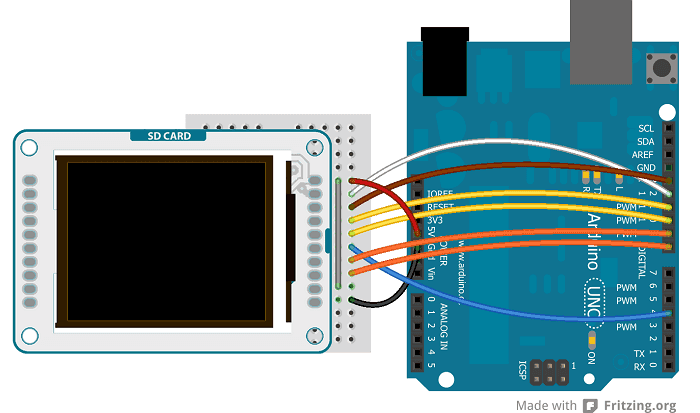
In this Arduino touch screen tutorial we will learn how to use TFT LCD Touch Screen with Arduino. You can watch the following video or read the written tutorial below.
For this tutorial I composed three examples. The first example is distance measurement using ultrasonic sensor. The output from the sensor, or the distance is printed on the screen and using the touch screen we can select the units, either centimeters or inches.
The next example is controlling an RGB LED using these three RGB sliders. For example if we start to slide the blue slider, the LED will light up in blue and increase the light as we would go to the maximum value. So the sliders can move from 0 to 255 and with their combination we can set any color to the RGB LED, but just keep in mind that the LED cannot represent the colors that much accurate.
The third example is a game. Actually it’s a replica of the popular Flappy Bird game for smartphones. We can play the game using the push button or even using the touch screen itself.
As an example I am using a 3.2” TFT Touch Screen in a combination with a TFT LCD Arduino Mega Shield. We need a shield because the TFT Touch screen works at 3.3V and the Arduino Mega outputs are 5 V. For the first example I have the HC-SR04 ultrasonic sensor, then for the second example an RGB LED with three resistors and a push button for the game example. Also I had to make a custom made pin header like this, by soldering pin headers and bend on of them so I could insert them in between the Arduino Board and the TFT Shield.
Here’s the circuit schematic. We will use the GND pin, the digital pins from 8 to 13, as well as the pin number 14. As the 5V pins are already used by the TFT Screen I will use the pin number 13 as VCC, by setting it right away high in the setup section of code.
As the code is a bit longer and for better understanding I will post the source code of the program in sections with description for each section. And at the end of this article I will post the complete source code.
I will use the UTFT and URTouch libraries made by Henning Karlsen. Here I would like to say thanks to him for the incredible work he has done. The libraries enable really easy use of the TFT Screens, and they work with many different TFT screens sizes, shields and controllers. You can download these libraries from his website, RinkyDinkElectronics.com and also find a lot of demo examples and detailed documentation of how to use them.
After we include the libraries we need to create UTFT and URTouch objects. The parameters of these objects depends on the model of the TFT Screen and Shield and these details can be also found in the documentation of the libraries.
So now I will explain how we can make the home screen of the program. With the setBackColor() function we need to set the background color of the text, black one in our case. Then we need to set the color to white, set the big font and using the print() function, we will print the string “Arduino TFT Tutorial” at the center of the screen and 10 pixels down the Y – Axis of the screen. Next we will set the color to red and draw the red line below the text. After that we need to set the color back to white, and print the two other strings, “by HowToMechatronics.com” using the small font and “Select Example” using the big font.
Now we need to make the buttons functional so that when we press them they would send us to the appropriate example. In the setup section we set the character ‘0’ to the currentPage variable, which will indicate that we are at the home screen. So if that’s true, and if we press on the screen this if statement would become true and using these lines here we will get the X and Y coordinates where the screen has been pressed. If that’s the area that covers the first button we will call the drawDistanceSensor() custom function which will activate the distance sensor example. Also we will set the character ‘1’ to the variable currentPage which will indicate that we are at the first example. The drawFrame() custom function is used for highlighting the button when it’s pressed. The same procedure goes for the two other buttons.
drawDistanceSensor(); // It is called only once, because in the next iteration of the loop, this above if statement will be false so this funtion won"t be called. This function will draw the graphics of the first example.
getDistance(); // Gets distance from the sensor and this function is repeatedly called while we are at the first example in order to print the lasest results from the distance sensor
So the drawDistanceSensor() custom function needs to be called only once when the button is pressed in order to draw all the graphics of this example in similar way as we described for the home screen. However, the getDistance() custom function needs to be called repeatedly in order to print the latest results of the distance measured by the sensor.
Ok next is the RGB LED Control example. If we press the second button, the drawLedControl() custom function will be called only once for drawing the graphic of that example and the setLedColor() custom function will be repeatedly called. In this function we use the touch screen to set the values of the 3 sliders from 0 to 255. With the if statements we confine the area of each slider and get the X value of the slider. So the values of the X coordinate of each slider are from 38 to 310 pixels and we need to map these values into values from 0 to 255 which will be used as a PWM signal for lighting up the LED. If you need more details how the RGB LED works you can check my particular tutorialfor that. The rest of the code in this custom function is for drawing the sliders. Back in the loop section we only have the back button which also turns off the LED when pressed.
In order the code to work and compile you will have to include an addition “.c” file in the same directory with the Arduino sketch. This file is for the third game example and it’s a bitmap of the bird. For more details how this part of the code work you can check my particular tutorial. Here you can download that file:
drawDistanceSensor(); // It is called only once, because in the next iteration of the loop, this above if statement will be false so this funtion won"t be called. This function will draw the graphics of the first example.
getDistance(); // Gets distance from the sensor and this function is repeatedly called while we are at the first example in order to print the lasest results from the distance sensor
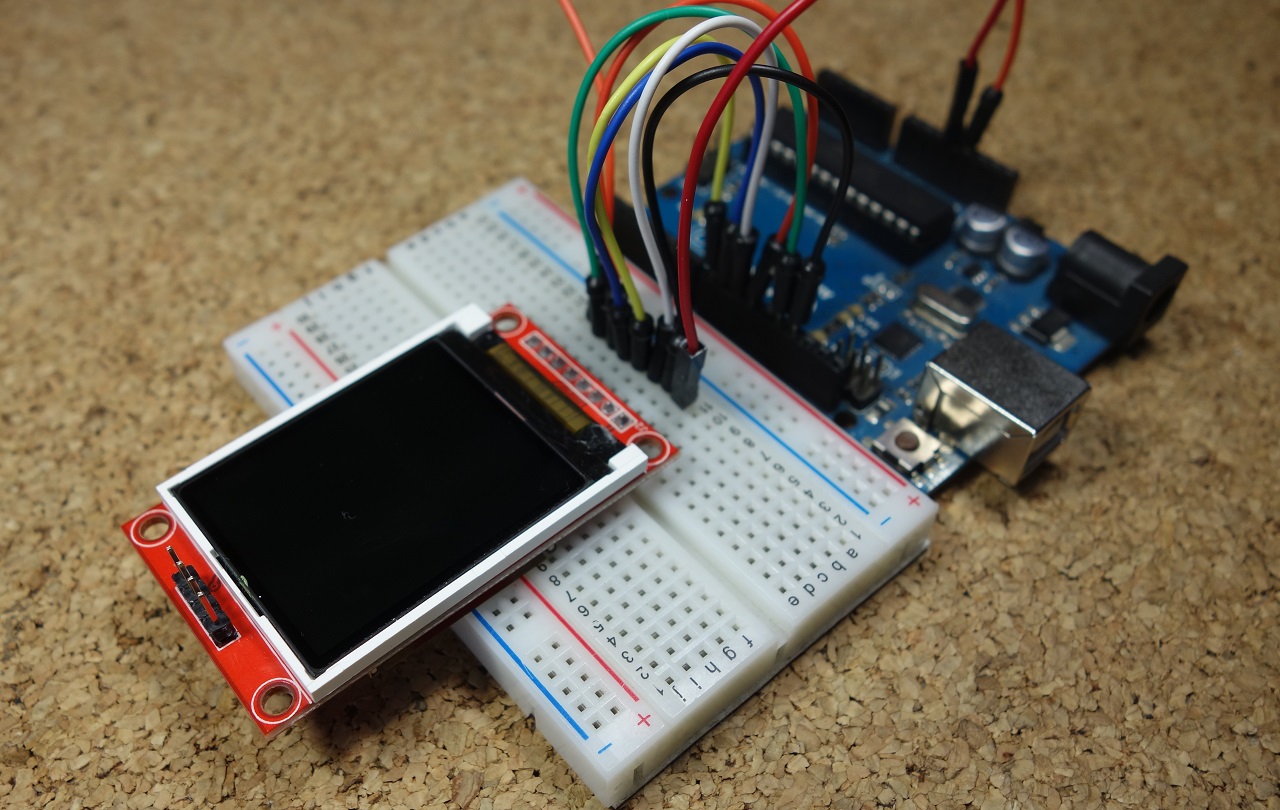
Voltage type: 5v or 3v voltage input voltage,input is selectable. Because TFT can only work under 3.3 V voltage, so when the input voltage VIN is 5V, need through the 3.3 V voltage regulator IC step down to 3.3V , when the input voltage of 3.3 V, you need to use the zero resistance make J2 short , is equivalent to not through the voltage regulator IC for module and power supply directly.
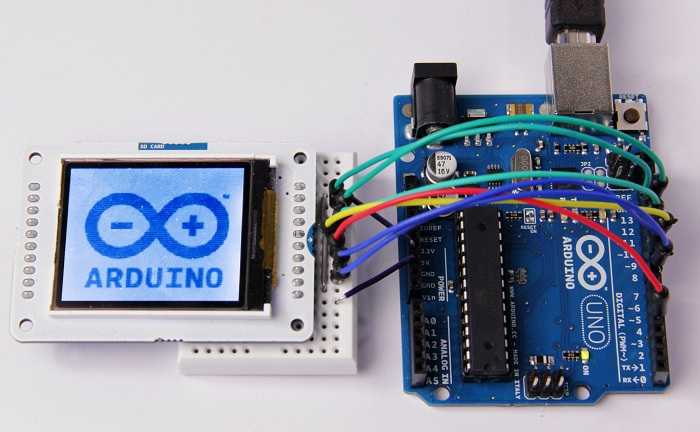
We covered the basics of accelerometer previously inUsing Arduino with Parts and Sensors – Accelerometer Part 1andUsing Arduino with Parts and Sensors – Accelerometer Part 2. Today we’ll be testing KX022-1020 accelerometer using TFT liquid crystal panel. We’ll discuss how to control the TFT LCD in more detail in the next article. In addition, we’ll further exploreArduino Create. For more information about Arduino Create, please refer back tothisarticle.
Let’s briefly review what accelerometer is. An accelerometer is a sensor that can detect the state of motion, such as “tilt,” “shock,” “vibration” and so forth. Accelerometers are classified into one axis, two axes, and three axes. For example, one axis detects one direction (vertical only); two axes detects two directions (vertical and horizontal); and three axes three directions (vertical, horizontal and height).
We’ll continue using Arduino Create Web Editor as we did in our lasttutorial. To add the library, you can upload the zip file by selecting it from “Libraries” on the left menu and clicking on “ADD ZIP LIBRARY.”
Now the sample program is working fine, let’s try to display the values on a 1.8 inch TFT LCD monitor. Although this TFT liquid crystal monitor has a resolution slightly smaller than 126 x 160 px, it’ll be quite useful when displaying numbers or letters with Arduino etc.
When using the TFT monitor, the connection method and the library used in the program may be different depending on the specification of each TFT monitor. The TFT monitor used in this tutorial is a monitorSainSmart ST7735R. In addition to Arduino, the monitor is also compatible with Raspberry.
In order to use the monitor to run the program in Arduino, we’ll have to modify the downloaded library a little bit.We’ll go over how to control the TFT LCD in more detail in the next article. Once everything is set, you will be able to output numerical values in the monitor as shown in the video below:
In the next part, we’ll create a simple device using the same accelerometer and TFT monitor. We’ll show how to create graphs and display the values obtained from the accelerometer on the TFT monitor.
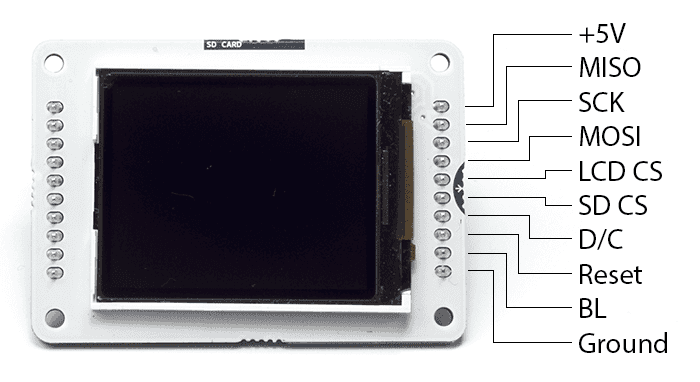
This guide is about DWIN HMI Touch Screen TFT LCD Display. HMI Means Human-Machine Interface. DWIN is specialized in making HMI Touch screen displays that are compatible with all microcontrollers like Arduino, STM32, PIC, and 8051 families of Microcontrollers.
This is a Getting Started tutorial with 7-inch DWIN HMI TFT LCD Display. We will see the architecture, features, board design, components, and specifications. We will also learn about the TTL & RS232 interfaces. Using the DGUS software you can create UI and with SD Card you can load the firmware on display memory.
On the LCD board, you can see the flip-open connector. Just flip open the connector and insert the FCC cable. Keep in mind that the blue ends should be on top. Now you can just press the lock so the FCC cable is locked.
One of the method to load the firmware to the T5L DWIN LCD Display is by using the SD Card. An SD Card of up to 16GB can be used to download the firmware files. We can easily insert the Micro SD card into the SD Card slot on the backside.
After copying the file, remove the SD Card from your computer and insert it into the SD Card slot of DWIN LCD Display. Then power the display using the USB Cable. The firmware downloading process will start automatically.
The next part of this tutorial includes creating UI and interfacing DWIN LCD Display with Arduino. For that you can follow the DWIN LCD Arduino Interfacing Guide.